|
1 |
| -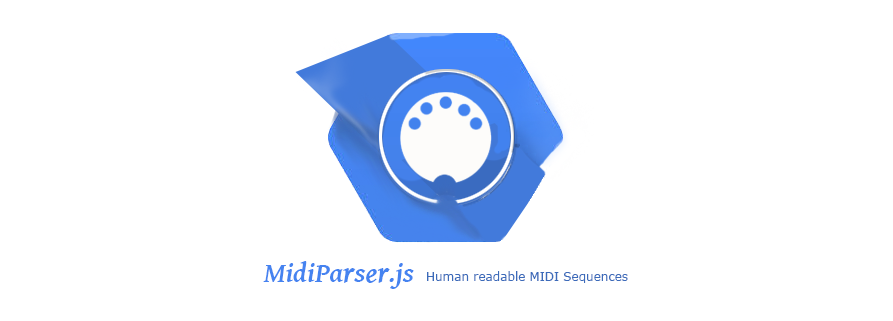 |
| 1 | +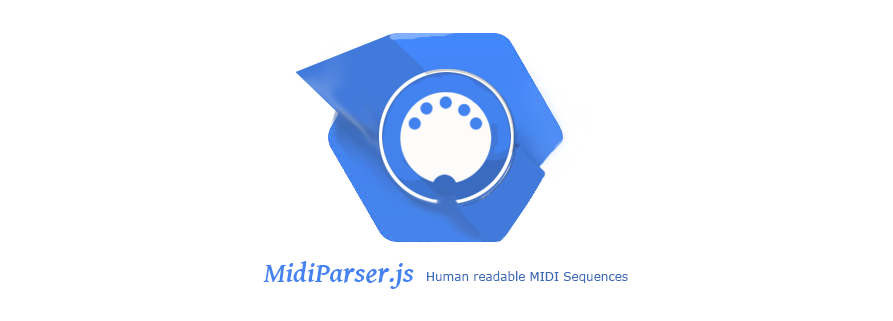 |
2 | 2 |
|
3 | 3 | # MidiParser.js
|
4 | 4 | [](https://github.com/colxi/midi-parser-js)
|
5 | 5 | [](https://github.com/colxi/midi-parser-js)
|
6 | 6 | [](https://www.npmjs.com/package/midi-parser-js)
|
7 | 7 |
|
8 |
| -MidiParser is a Javascript **Binary MIDI file** reader for the browser and Node which converts the binary data structure to **a JSON object**, making it much easier to iterate over and interact with. |
| 8 | +MidiParser is a Javascript **Binary MIDI file** reader for the browser and Node which converts a MIDI binary data structure to **a JSON object**, making it much easier to iterate over and interact with. |
9 | 9 |
|
10 |
| -> **It accepts ```BASE64``` encoded Midi data, or the resulting ```UINT8``` arrayBuffer, obtained after reading a raw .mid binary. It also, can automatically handle files selected with a ```FileInput Element``` in the browser.** |
| 10 | +- Tiny and dependency free |
| 11 | +- Browser & Node Compatible |
| 12 | +- Supported data input : |
| 13 | + - ```BASE64``` encoded Midi data |
| 14 | + - ```UINT8``` arrayBuffer, obtained when reading or fetching a .mid binary. |
| 15 | + - ```FileInput Element``` in the Browser |
| 16 | +- Custom Midi Messages |
11 | 17 |
|
12 | 18 |
|
13 |
| -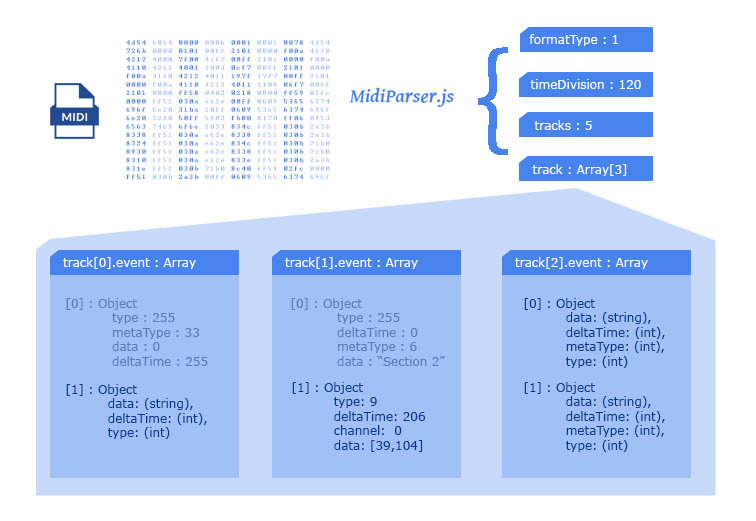 |
14 | 19 |
|
| 20 | +## Example |
15 | 21 |
|
16 |
| -## Syntax: |
| 22 | +A simple example parsing a MIDI file in Node ... |
| 23 | +```javascript |
| 24 | +let midiParser = require('midi-parser-js'); |
| 25 | +let fs = require('fs') |
| 26 | + |
| 27 | +// read a .mid binary (as base64) |
| 28 | +fs.readFile('./test.mid', 'base64', function (err,data) { |
| 29 | + // Parse the obtainer base64 string ... |
| 30 | + var midiArray = midiParser.parse(data); |
| 31 | + // done! |
| 32 | + console.log(midiArray); |
| 33 | +}); |
| 34 | +``` |
| 35 | + |
| 36 | +Example in Browser... |
| 37 | +```html |
| 38 | +<script type="module"> |
| 39 | + import {MidiParser} from 'midi-parser.js' |
| 40 | + // select the INPUT element that will handle |
| 41 | + // the file selection. |
| 42 | + let source = document.getElementById('filereader'); |
| 43 | + // provide the File source and a callback function |
| 44 | + MidiParser.parse( source, function(obj){ |
| 45 | + console.log(obj); |
| 46 | + }); |
| 47 | +</script> |
| 48 | +<input type="file" id="filereader"/> |
| 49 | +``` |
| 50 | +If you want to see it in action, you can test it [Here](https://colxi.info/midi-parser-js/test/test-es6-import.html) |
17 | 51 |
|
| 52 | +## Syntax: |
18 | 53 |
|
19 |
| -> MidiParser.parse( input [, callback] ); |
20 | 54 |
|
21 |
| -- **input** : Accepts any of the supported Data Sources (```FileInputElement```|```uint8Array```|```base64String```) |
| 55 | +```javascript |
| 56 | + MidiParser.parse( input [, callback] ); |
| 57 | +``` |
| 58 | +**- input** : Accepts any of the supported Data Sources : `FileInputElement | uint8Array | base64String` |
22 | 59 |
|
23 |
| -- **callback** : Function to handle the parsed data. Only required when input is a FileInputElement. |
| 60 | +**- callback** : Callback to be executed when data is parsed. Only required when input is a `FileInputElement`. |
24 | 61 |
|
25 | 62 |
|
26 | 63 |
|
27 | 64 | ---
|
28 | 65 |
|
29 |
| -### Handle Custom messages ( sysEx, non-standard...) |
| 66 | +## Handle Custom messages ( sysEx, non-standard...) |
30 | 67 |
|
31 | 68 | By default, the library ignores the sysEx, and non-standard messages, simply converting their values to integers (when possible).
|
32 | 69 | However you can provide a custom hook function to be executed when any non-standard message is found, and process it by your own, returning the resulting value.
|
33 | 70 |
|
34 | 71 |
|
35 |
| -> MidiParser.customInterpreter = function( msgType, arrayBuffer, metaEventLength){ /** your code ** / } |
| 72 | +```javascript |
| 73 | +MidiParser.customInterpreter = function( msgType, arrayBuffer, metaEventLength){ /* your code */ } |
| 74 | +``` |
36 | 75 |
|
37 |
| -- **msgType** : Hex value of the message type |
| 76 | +**- msgType** : Hex value of the message type |
38 | 77 |
|
39 |
| -- **arrayBuffer** : Dataview of the midi data. You have to extract your value/s from it, moving the pointer as needed. |
| 78 | +**- arrayBuffer** : Dataview of the midi data. You have to extract your value/s from it, moving the pointer as needed. |
40 | 79 |
|
41 |
| -- **metaEventLength** : A length greater than 0 indicates a received message |
| 80 | +**- metaEventLength** : A length greater than 0 indicates a received message |
42 | 81 |
|
43 | 82 | > If you want the default action to be executed, return **false**
|
44 | 83 |
|
45 | 84 |
|
46 |
| -## Parsed Data Structure : |
| 85 | +## Output JSON anatomy : |
| 86 | + |
| 87 | +The returned JSON object contains all the attributes of the MIDI file (format type, time division, track count... ) as properties. The tracks and the MIDI events related to each track are container inside the `track` property. |
47 | 88 |
|
| 89 | +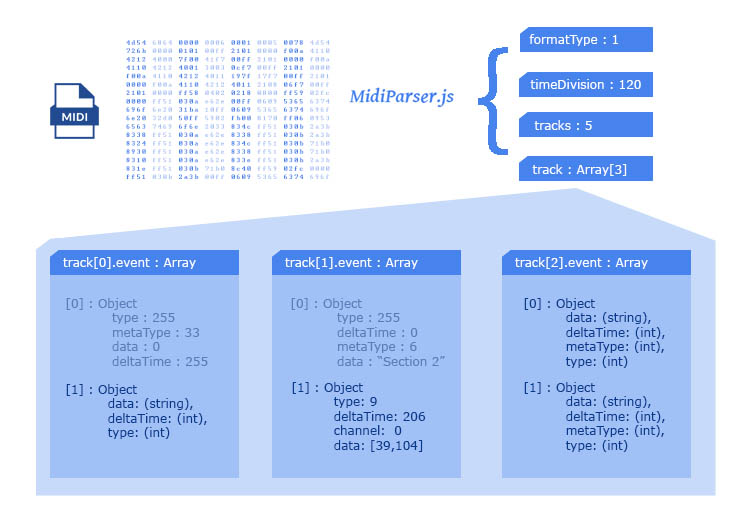 |
| 90 | + |
| 91 | + |
| 92 | +The following JSON object represents a MIDI file with **3 tracks** and **4 events** in **Track 0** |
48 | 93 |
|
49 | 94 | ```javascript
|
50 |
| -Output_Object{ |
51 |
| - formatType: 0|1|2, // Midi format type |
52 |
| - timeDivision: (int), // song tempo (bpm) |
53 |
| - tracks: (int), // total tracks count |
54 |
| - track: Array[ |
55 |
| - [0]: Object{ // TRACK 1! |
56 |
| - event: Array[ // Midi events in track 1 |
57 |
| - [0] : Object{ // EVENT 1 |
58 |
| - data: (string), |
59 |
| - deltaTime: (int), |
60 |
| - metaType: (int), |
61 |
| - type: (int), |
62 |
| - }, |
63 |
| - [1] : Object{...}, // EVENT 2 |
64 |
| - [2] : Object{...}, // EVENT 3 |
65 |
| - // ... |
66 |
| - ] |
67 |
| - }, |
68 |
| - [1] : Object{...}, // TRACK 2 |
69 |
| - [2] : Object{...}, // TRACK 3 |
70 |
| - // ... |
71 |
| - ] |
| 95 | +outputObject{ |
| 96 | +....formatType: 0|1|2, // Midi format type |
| 97 | +....timeDivision: (int), // song tempo (bpm) |
| 98 | +....tracks: (int), // total tracks count |
| 99 | +....track: Array[ |
| 100 | +........[0]: Object{ // TRACK 1! |
| 101 | +............event: Array[ // Midi events in track 1 |
| 102 | +................[0] : Object{ // EVENT 1 |
| 103 | +....................data: (string), |
| 104 | +....................deltaTime: (int), |
| 105 | +....................metaType: (int), |
| 106 | +....................type: (int) |
| 107 | +................}, |
| 108 | +................[1] : Object{...}, // EVENT 2 |
| 109 | +................[2] : Object{...}, // EVENT 3 |
| 110 | +................[3] : Object{...} // EVENT 4 |
| 111 | +............] |
| 112 | +........}, |
| 113 | +........[1] : Object{...}, // TRACK 2 |
| 114 | +........[2] : Object{...} // TRACK 3 |
| 115 | +....] |
72 | 116 | }
|
73 | 117 | ```
|
74 |
| -The data from **Event 12** of **Track 2** can be read like this: |
| 118 | +If you want to read the data from **Event 2** of **Track 0** , you should use the following keypath : |
| 119 | + |
75 | 120 | ```javascript
|
76 |
| -Output_Object.track[2].event[12].data; |
| 121 | +outputObject.track[0].event[2].data; |
77 | 122 | ```
|
| 123 | +--- |
78 | 124 |
|
79 |
| -## Installation : |
| 125 | +## Distribution & Installation : |
| 126 | + |
| 127 | +The following distribution channels are available : |
80 | 128 |
|
81 |
| -The following methods are available to use MidiParser.js : |
82 | 129 |
|
| 130 | +**- NPM** : Install using the following command : |
83 | 131 |
|
84 |
| -- Include this library in your HTML head using the CDN : |
| 132 | +```bash |
| 133 | + $ npm install midi-parser-js -s |
| 134 | +``` |
85 | 135 |
|
86 |
| -> https://gitcdn.link/repo/colxi/midi-parser-js/master/src/midi-parser.js |
| 136 | +**- GIT** : You can clone the repository : |
| 137 | +```bash |
| 138 | + $ git clone https://github.com/colxi/midi-parser-js.git |
| 139 | +``` |
87 | 140 |
|
88 |
| -- Use NPM to install the package : |
| 141 | +**-ZIP** : Or download the package in a ZIP file from |
| 142 | +> [GITHUB LATEST PACKAGE RELEASE PAGE](https://github.com/colxi/midi-parser-js/releases/latest) |
89 | 143 |
|
90 |
| -> $ npm install midi-parser-js |
91 | 144 |
|
92 |
| -- Clone the repository from GITHUB : |
| 145 | +**-CDN** : Include the latest release of this library in your HTML head using the CDN : |
| 146 | +> Warning : Not recommended for production enviroments! |
93 | 147 |
|
94 |
| -> $ git clone https://github.com/colxi/midi-parser-js.git |
| 148 | +```html |
| 149 | +<script src="https://colxi.info/midi-parser-js/src/main.js"></script> |
| 150 | +``` |
| 151 | +## Importing |
| 152 | +This package is shipped with support to Node CommonJS and ES6 Modules. Use the appropiate method accoordintg to your enviroment. |
95 | 153 |
|
| 154 | +```javascript |
| 155 | + // ES6 Module Import : |
| 156 | + import {MidiParser} from './midi-parser.js'; |
96 | 157 |
|
| 158 | + // CommonJS Node Import : |
| 159 | + let MidiParser = require('midi-parser-js'); |
| 160 | +``` |
97 | 161 |
|
98 |
| -## MIDI File Format Specifications : |
| 162 | +--- |
| 163 | +## Bonus : MIDI File Format Specifications : |
99 | 164 |
|
100 | 165 | MIDI Binary Encoding Specifications in https://github.com/colxi/midi-parser-js/wiki/MIDI-File-Format-Specifications
|
101 | 166 |
|
0 commit comments