|
| 1 | +3367\. Maximize Sum of Weights after Edge Removals |
| 2 | + |
| 3 | +Hard |
| 4 | + |
| 5 | +There exists an **undirected** tree with `n` nodes numbered `0` to `n - 1`. You are given a 2D integer array `edges` of length `n - 1`, where <code>edges[i] = [u<sub>i</sub>, v<sub>i</sub>, w<sub>i</sub>]</code> indicates that there is an edge between nodes <code>u<sub>i</sub></code> and <code>v<sub>i</sub></code> with weight <code>w<sub>i</sub></code> in the tree. |
| 6 | + |
| 7 | +Your task is to remove _zero or more_ edges such that: |
| 8 | + |
| 9 | +* Each node has an edge with **at most** `k` other nodes, where `k` is given. |
| 10 | +* The sum of the weights of the remaining edges is **maximized**. |
| 11 | + |
| 12 | +Return the **maximum** possible sum of weights for the remaining edges after making the necessary removals. |
| 13 | + |
| 14 | +**Example 1:** |
| 15 | + |
| 16 | +**Input:** edges = [[0,1,4],[0,2,2],[2,3,12],[2,4,6]], k = 2 |
| 17 | + |
| 18 | +**Output:** 22 |
| 19 | + |
| 20 | +**Explanation:** |
| 21 | + |
| 22 | +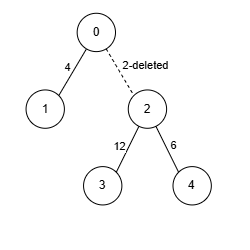 |
| 23 | + |
| 24 | +* Node 2 has edges with 3 other nodes. We remove the edge `[0, 2, 2]`, ensuring that no node has edges with more than `k = 2` nodes. |
| 25 | +* The sum of weights is 22, and we can't achieve a greater sum. Thus, the answer is 22. |
| 26 | + |
| 27 | +**Example 2:** |
| 28 | + |
| 29 | +**Input:** edges = [[0,1,5],[1,2,10],[0,3,15],[3,4,20],[3,5,5],[0,6,10]], k = 3 |
| 30 | + |
| 31 | +**Output:** 65 |
| 32 | + |
| 33 | +**Explanation:** |
| 34 | + |
| 35 | +* Since no node has edges connecting it to more than `k = 3` nodes, we don't remove any edges. |
| 36 | +* The sum of weights is 65. Thus, the answer is 65. |
| 37 | + |
| 38 | +**Constraints:** |
| 39 | + |
| 40 | +* <code>2 <= n <= 10<sup>5</sup></code> |
| 41 | +* `1 <= k <= n - 1` |
| 42 | +* `edges.length == n - 1` |
| 43 | +* `edges[i].length == 3` |
| 44 | +* `0 <= edges[i][0] <= n - 1` |
| 45 | +* `0 <= edges[i][1] <= n - 1` |
| 46 | +* <code>1 <= edges[i][2] <= 10<sup>6</sup></code> |
| 47 | +* The input is generated such that `edges` form a valid tree. |
0 commit comments